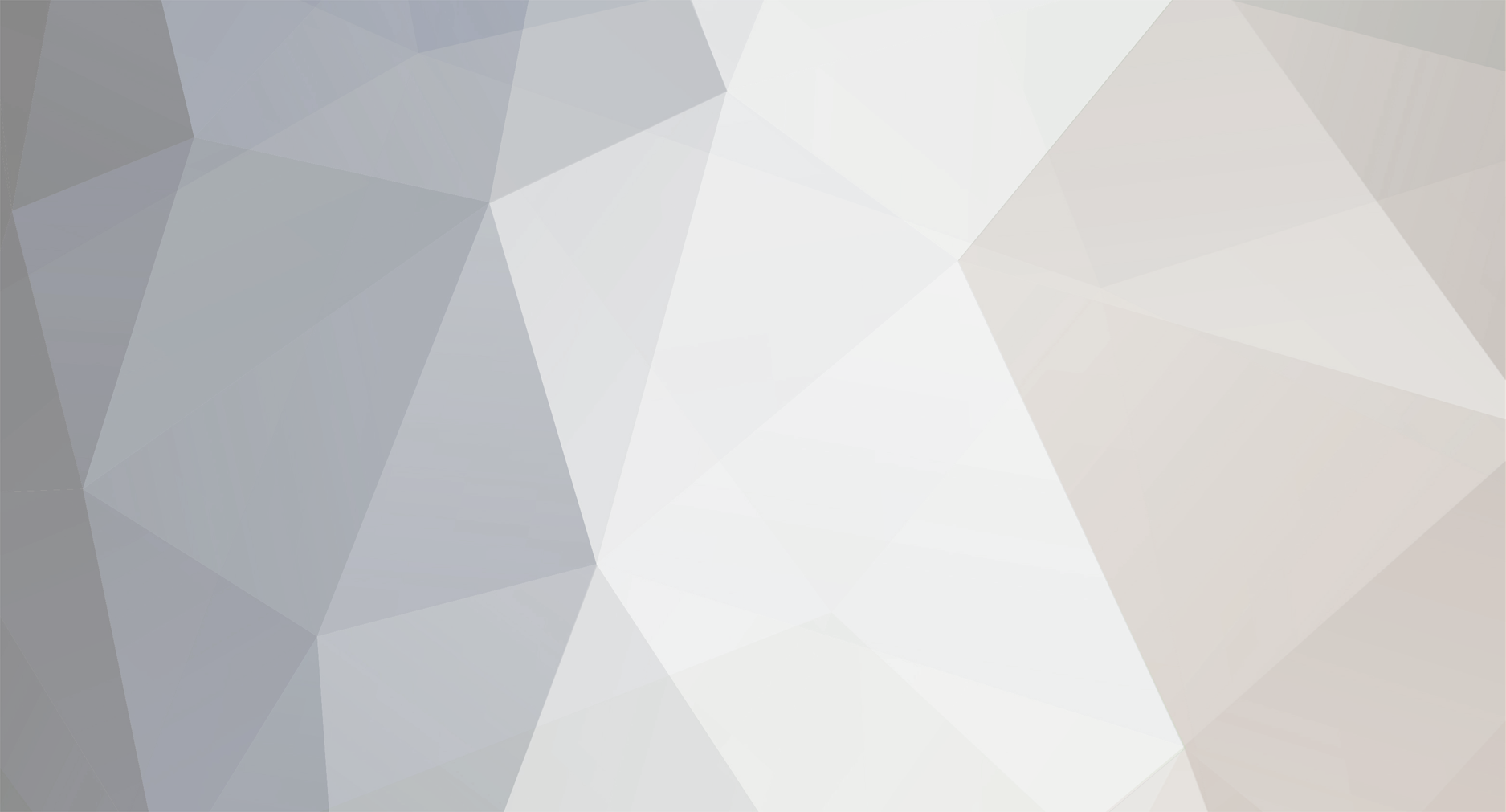
lenhao
-
Total de itens
11 -
Registro em
-
Última visita
Posts postados por lenhao
-
-
<?php
// constants used by class
define('MYSQL_TYPES_NUMERIC', 'int real ');
define('MYSQL_TYPES_DATE', 'datetime timestamp year date time ');
define('MYSQL_TYPES_STRING', 'string blob ');require 'configs.php'; if (!defined('_VALID_'))header("Location: index");
class db_class {
var $last_error; // holds the last error. Usually mysql_error()
var $last_query; // holds the last query executed.
var $row_count; // holds the last number of rows from a select
private $server = ''; //database server
private $pw = ''; //database login password
private $database = ''; //database name
private $pre = ''; //table prefix
private $err_email = '';
/*
// private váriaveis
private $USER_TABLE='';
private $NEWS_TABLE='';
private $ADMIN_TABLE='';
private $CONFIG_TABLE='';
*/
private $errlog = true; // true or false
private $error = '';
private $errno = 0;
var $link_id; // current/last database link identifier
var $auto_slashes; // the class will add/strip slashes when it can
public function db_class() {
$this->server=DB_HOST;
$this->user=DB_USER;
$this->pw=DB_PASS;
$this->database=DB_NAME;
$this->pre=DB_TABLE;
/*
// private váriaveis
$this->USER_TABLE=TABLE_USER;
$this->NEWS_TABLE=TABLE_NEWS;
$this->ADMIN_TABLE=TABLE_ADMIN;
$this->CONFIG_TABLE=CONFIG_TABLE;
*/
$this->auto_slashes = true;
}public function connect($new_link=false, $persistant=false) {
if ($persistant)
$this->link_id =@mysql_connect($this->server,$this->user,$this->pw,$new_link);
else
$this->link_id =@mysql_connect($this->server,$this->user,$this->pw,$new_link);
if (!$this->link_id) {
$this->last_error = mysql_error();
$this->print_last_error("Connection close failed.");
return false;
}
if(!@mysql_select_db($this->database, $this->link_id)) {//no database
$this->last_error = mysql_error();
}
if (!$this->select_db($this->database, $this->link_id)) return false;
return $this->link_id; // success
}
public function close() {
if(!mysql_close($this->link_id)){
$this->last_error = "Connection close failed.";
}
}
private function escape($string) {
if(get_magic_quotes_gpc()) $string = stripslashes($string);
return mysql_real_escape_string($string);
}
function select_db($db='') {
if (!empty($db)) $this->db = $db;
if (!mysql_select_db($this->db)) {
$this->last_error = mysql_error();
return false;
}
return true;
}
function select($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$this->row_count = mysql_num_rows($r);
return $r;
}
function select_array($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$this->row_count = mysql_fetch_array($r);
return $r;
}
public function query($query) {
$r = mysql_query($sql);
if (!$r) {
$r->result = mysql_query($query);
} else {
$r->result = null;
}
}
function select_one($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
if (mysql_num_rows($r) > 1) {
$this->last_error = "Your query in function select_one() returned more that one result.";
return false;
}
if (mysql_num_rows($r) < 1) {
$this->last_error = "Your query in function select_one() returned no results.";
return false;
}
$ret = mysql_result($r, 0);
mysql_free_result($r);
if ($this->auto_slashes) return stripslashes($ret);
else return $ret;
}
function get_row($result, $type='MYSQL_BOTH') {
if (!$result) {
$this->last_error = "Invalid resource identifier passed to get_row() function.";
return false;
}
if ($type == 'MYSQL_ASSOC') $row = mysql_fetch_array($result, MYSQL_ASSOC);
if ($type == 'MYSQL_NUM') $row = mysql_fetch_array($result, MYSQL_NUM);
if ($type == 'MYSQL_BOTH') $row = mysql_fetch_array($result, MYSQL_BOTH);
if (!$row) return false;
if ($this->auto_slashes) {
foreach ($row as $key => $value) {
$row[$key] = stripslashes($value);
}
}
return $row;
}
function dump_query($data,$data2,$data3) {
$r = $this->select("SELECT * FROM $data WHERE $data2=$data3");
if (!$r) return false;
echo "<table cellpadding=\"3\" cellspacing=\"1\" border=\"0\" width=\"100%\">\n";
$i = 0;
while ($row = mysql_fetch_assoc($r)) {
if ($i == 0) {
foreach ($row as $col => $value) {
echo "<td bgcolor=\"#E6E5FF\"><span style=\"font-face: sans-serif; font-size: 9pt; font-weight: bold;\">$col</span></td>\n";
}
echo "</tr>\n";
}
$i++;
if ($i % 2 == 0) $bg = '#E3E3E3';
else $bg = '#F3F3F3';
echo "<tr>\n";
foreach ($row as $value) {
echo "<td bgcolor=\"$bg\"><span style=\"font-face: sans-serif; font-size: 9pt;\">$value</span></td>\n";
}
echo "</tr>\n";
}
echo "</table></div>\n";
}
function insert_sql($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$id = mysql_insert_id();
if ($id == 0) return true;
else return $id;
}
function update_sql($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$rows = mysql_affected_rows();
if ($rows == 0) return true;
else return $rows;
}
function insert_array($table, $data) {
if (empty($data)) {
$this->last_error = "You must pass an array to the insert_array() function.";
return false;
}
$cols = '(';
$values = '(';
foreach ($data as $key=>$value) {
$cols .= "$key,";
$col_type = $this->get_column_type($table, $key);
if (!$col_type) return false;
if (is_null($value)) {
$values .= "NULL,";
}
elseif (substr_count(MYSQL_TYPES_NUMERIC, "$col_type ")) {
$values .= "$value,";
}
elseif (substr_count(MYSQL_TYPES_DATE, "$col_type ")) {
$value = $this->sql_date_format($value, $col_type);
$values .= "'$value',";
}
elseif (substr_count(MYSQL_TYPES_STRING, "$col_type ")) {
if ($this->auto_slashes) $value = addslashes($value);
$values .= "'$value',";
}
}
$cols = rtrim($cols, ',').')';
$values = rtrim($values, ',').')';
$sql = "INSERT INTO $table $cols VALUES $values";
return $this->insert_sql($sql);
}
function update_array($table, $data, $condition) {
if (empty($data)) {
$this->last_error = "You must pass an array to the update_array() function.";
return false;
}
$sql = "UPDATE $table SET";
foreach ($data as $key=>$value) {
$sql .= " $key=";
$col_type = $this->get_column_type($table, $key);
if (!$col_type) return false;
if (is_null($value)) {
$sql .= "NULL,";
}
elseif (substr_count(MYSQL_TYPES_NUMERIC, "$col_type ")) {
$sql .= "$value,";
}
elseif (substr_count(MYSQL_TYPES_DATE, "$col_type ")) {
$value = $this->sql_date_format($value, $col_type);
$sql .= "'$value',";
}
elseif (substr_count(MYSQL_TYPES_STRING, "$col_type ")) {
if ($this->auto_slashes) $value = addslashes($value);
$sql .= "'$value',";
}
}
$sql = rtrim($sql, ',');
if (!empty($condition)) $sql .= "WHERE $condition";
return $this->update_sql($sql);
}
function execute_file ($file) {
if (!file_exists($file)) {
$this->last_error = "The file $file does not exist.";
return false;
}
$str = file_get_contents($file);
if (!$str) {
$this->last_error = "Unable to read the contents of $file.";
return false;
}
$this->last_query = $str;
$sql = explode(';', $str);
foreach ($sql as $query) {
if (!empty($query)) {
$r = mysql_query($query);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
}
}
return true;
}
function get_column_type($table, $column) {
$r = mysql_query("SELECT $column FROM $table");
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$ret = mysql_field_type($r, 0);
if (!$ret) {
$this->last_error = "Unable to get column information on $table.$column.";
mysql_free_result($r);
return false;
}
mysql_free_result($r);
return $ret;
}
function sql_date_format($value) {
if (gettype($value) == 'string') $value = strtotime($value);
return date('Y-m-d H:i:s', $value);
}
function print_last_error($show_query=true) {
?>
<div style="border: 1px solid red; font-size: 9pt; font-family: monospace; color: red; padding: .5em; margin: 8px; background-color: #FFE2E2">
<span style="font-weight: bold">db.class.php Error:</span><br><?= $this->last_error ?>
</div>
<?
if ($show_query && (!empty($this->last_query))) {
$this->print_last_query();
}
}
public function get_link_id() {
return $this->link_id;
}
function print_last_query() {
?>
<div style="border: 1px solid blue; font-size: 9pt; font-family: monospace; color: blue; padding: .5em; margin: 8px; background-color: #E6E5FF">
<span style="font-weight: bold">Last SQL Query:</span><br><?= str_replace("\n", '<br>', $this->last_query) ?>
</div>
<?
}
function filter($data) {
$this->connect();
$data = trim(htmlentities(strip_tags($data)));
if (get_magic_quotes_gpc())
$data = stripslashes($data);
$data = mysql_real_escape_string($data);
return $data;
}
function page () {
ob_start();
session_start('WebATS');
include ("inc/htmltags.php");
include ("inc/class.content.php");
$Content = new Content();
$Content->ShowContent(PAGE);
echo '<title>'.$title.' - '.utf8_decode(PAGENAME).'</title>';
include ("inc/footer.php");
}
function account ($data) {
if($data == 'createaccount'){
define('_PAGETYPEREGISTER_','active');
} elseif ($data == 'login'){
define('_PAGETYPELOGIN_','active');
}elseif($data == ''){
define('_PAGETYPEREGISTER_','active');
}elseif($data == 'forgot_password'){
define('_PAGETYPEFORGOT_','active');
}elseif($data == 'active'){
define('_PAGETYPEACTIVE_','active');
}
}
}
?>está assim agora, necessito ajuda, muito obrigado!!
-
como você resolveu?? estou com o mesmo problema, necessito de ajuda
-
revisei agora novamente e não encontrei erro algum, necessito de ajudo, por favor. Estou ficando louco
a unica que coisa que me vem na cabeça é algum ponto e virgula, mas não sei aonde colocar, nem procurar, necessito de ajuda, Muito obrigado!!
-
já olhei, já reli, li umas 5 vezes e tudo bate, aparentemente não érro de aspas, virgulas, ou chaves/parenteses, o que mais pode ser ?
-
eu capitei a ideia, e apliquei no arquivo de cabo a rabo, e continua dando o mesmo erro, qual o próximo passo?
esta td mt confuso. Agradeço pela ajuda e dedicação, obrigado!
-
também, logo nas primeiras linhas, isso é tudo muito confuso, como alinho essas chaves? :
public function db_class() {
$this->server=DB_HOST;
$this->user=DB_USER;
$this->pw=DB_PASS;
$this->database=DB_NAME;
$this->pre=DB_TABLE;
/*
// private váriaveis
$this->USER_TABLE=TABLE_USER;
$this->NEWS_TABLE=TABLE_NEWS;
$this->ADMIN_TABLE=TABLE_ADMIN;
$this->CONFIG_TABLE=CONFIG_TABLE;
*/
$this->auto_slashes = true;
}me ajude, nesses primeiros que postei, que assim eu tenho uma ideia de como aplicar no arquivo inteiro:
if (!$this->link_id) {
$this->last_error = mysql_error();
$this->print_last_error("Connection close failed.");
return false;
}
if(!@mysql_select_db($this->database, $this->link_id)) {//no database
$this->last_error = mysql_error();
}
if (!$this->select_db($this->database, $this->link_id)) return false;
return $this->link_id; // success
} -
e quando as chaves vem logo após as palavras, por exemplo:
if ($show_query && (!empty($this->last_query))) {
$this->print_last_query();
}
}
public function get_link_id() {
return $this->link_id;
}como se alinha isso?
-
eu li, reli, e realmente não entendo nada disso, já procurei, parenteses, ;, chaves, aspas e não encontrei nenhum erro, sou um ignorante no assunto e gostaria que me ajudasse no scripr, muito obrigado pela atenção, desde já!
-
Muito obrigado, mas não está muito explícito para mim, não consigo ainda achar o erro, pois pode ser qualquer caractere em qualquer linha anterior, sou um ignorante nesse assunto. Necessito de ajuda! Muito obrigado
-
Olá, atualmente, estou tentando colocar um site on através do xampp, os arquivos já vieram prontos e não manjo nada de script/php e quando tento entrar no localhost, aparece o seguinte erro: Parse error: syntax error, unexpected $end in C:\xampp\htdocs\inc\db.class.php on line 397
vou postar o script inteiro, gostaria muito de ajuda, muito obrigado!!
<?php
// constants used by class
define('MYSQL_TYPES_NUMERIC', 'int real ');
define('MYSQL_TYPES_DATE', 'datetime timestamp year date time ');
define('MYSQL_TYPES_STRING', 'string blob ');require 'configs.php'; if (!defined('_VALID_'))header("Location: index");
class db_class {
var $last_error; // holds the last error. Usually mysql_error()
var $last_query; // holds the last query executed.
var $row_count; // holds the last number of rows from a select
private $server = ''; //database server
private $pw = ''; //database login password
private $database = ''; //database name
private $pre = ''; //table prefix
private $err_email = ADMIN_EMAIL;
/*
// private váriaveis
private $USER_TABLE = '';
private $NEWS_TABLE ='';
private $ADMIN_TABLE='';
private $CONFIG_TABLE='';
*/
private $errlog = true; // true or false
private $error = '';
private $errno = 0;
var $link_id; // current/last database link identifier
var $auto_slashes; // the class will add/strip slashes when it can
public function db_class() {
$this->server=DB_HOST;
$this->user=DB_USER;
$this->pw=DB_PASS;
$this->database=DB_NAME;
$this->pre=DB_TABLE;
/*
// private váriaveis
$this->USER_TABLE=TABLE_USER;
$this->NEWS_TABLE=TABLE_NEWS;
$this->ADMIN_TABLE=TABLE_ADMIN;
$this->CONFIG_TABLE=CONFIG_TABLE;
*/
$this->auto_slashes = true;
}public function connect($new_link=false, $persistant=false) {
if ($persistant)
$this->link_id =@mysql_connect($this->server,$this->user,$this->pw,$new_link);
else
$this->link_id =@mysql_connect($this->server,$this->user,$this->pw,$new_link);
if (!$this->link_id) {
$this->last_error = mysql_error();
$this->print_last_error("Connection close failed.");
return false;
}
if(!@mysql_select_db($this->database, $this->link_id)) {//no database
$this->last_error = mysql_error();
}
if (!$this->select_db($this->database, $this->link_id)) return false;
return $this->link_id; // success
}
public function close() {
if(!mysql_close($this->link_id)){
$this->last_error = "Connection close failed.";
}
}
private function escape($string) {
if(get_magic_quotes_gpc()) $string = stripslashes($string);
return mysql_real_escape_string($string);
}
function select_db($db='') {
if (!empty($db)) $this->db = $db;
if (!mysql_select_db($this->db)) {
$this->last_error = mysql_error();
return false;
}
return true;
}
function select($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$this->row_count = mysql_num_rows($r);
return $r;
}
function select_array($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$this->row_count = mysql_fetch_array($r);
return $r;
}
public function query($query) {
$r = mysql_query($sql);
if (!$r) {
$r->result = mysql_query($query);
} else {
$r->result = null;
}
}
function select_one($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
if (mysql_num_rows($r) > 1) {
$this->last_error = "Your query in function select_one() returned more that one result.";
return false;
}
if (mysql_num_rows($r) < 1) {
$this->last_error = "Your query in function select_one() returned no results.";
return false;
}
$ret = mysql_result($r, 0);
mysql_free_result($r);
if ($this->auto_slashes) return stripslashes($ret);
else return $ret;
}
function get_row($result, $type='MYSQL_BOTH') {
if (!$result) {
$this->last_error = "Invalid resource identifier passed to get_row() function.";
return false;
}
if ($type == 'MYSQL_ASSOC') $row = mysql_fetch_array($result, MYSQL_ASSOC);
if ($type == 'MYSQL_NUM') $row = mysql_fetch_array($result, MYSQL_NUM);
if ($type == 'MYSQL_BOTH') $row = mysql_fetch_array($result, MYSQL_BOTH);
if (!$row) return false;
if ($this->auto_slashes) {
foreach ($row as $key => $value) {
$row[$key] = stripslashes($value);
}
}
return $row;
}
function dump_query($data,$data2,$data3) {
$r = $this->select("SELECT * FROM $data WHERE $data2=$data3");
if (!$r) return false;
echo "<table cellpadding=\"3\" cellspacing=\"1\" border=\"0\" width=\"100%\">\n";
$i = 0;
while ($row = mysql_fetch_assoc($r)) {
if ($i == 0) {
foreach ($row as $col => $value) {
echo "<td bgcolor=\"#E6E5FF\"><span style=\"font-face: sans-serif; font-size: 9pt; font-weight: bold;\">$col</span></td>\n";
}
echo "</tr>\n";
}
$i++;
if ($i % 2 == 0) $bg = '#E3E3E3';
else $bg = '#F3F3F3';
echo "<tr>\n";
foreach ($row as $value) {
echo "<td bgcolor=\"$bg\"><span style=\"font-face: sans-serif; font-size: 9pt;\">$value</span></td>\n";
}
echo "</tr>\n";
}
echo "</table></div>\n";
}
function insert_sql($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$id = mysql_insert_id();
if ($id == 0) return true;
else return $id;
}
function update_sql($sql) {
$this->last_query = $sql;
$r = mysql_query($sql);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$rows = mysql_affected_rows();
if ($rows == 0) return true;
else return $rows;
}
function insert_array($table, $data) {
if (empty($data)) {
$this->last_error = "You must pass an array to the insert_array() function.";
return false;
}
$cols = '(';
$values = '(';
foreach ($data as $key=>$value) {
$cols .= "$key,";
$col_type = $this->get_column_type($table, $key);
if (!$col_type) return false;
if (is_null($value)) {
$values .= "NULL,";
}
elseif (substr_count(MYSQL_TYPES_NUMERIC, "$col_type ")) {
$values .= "$value,";
}
elseif (substr_count(MYSQL_TYPES_DATE, "$col_type ")) {
$value = $this->sql_date_format($value, $col_type);
$values .= "'$value',";
}
elseif (substr_count(MYSQL_TYPES_STRING, "$col_type ")) {
if ($this->auto_slashes) $value = addslashes($value);
$values .= "'$value',";
}
}
$cols = rtrim($cols, ',').')';
$values = rtrim($values, ',').')';
$sql = "INSERT INTO $table $cols VALUES $values";
return $this->insert_sql($sql);
}
function update_array($table, $data, $condition) {
if (empty($data)) {
$this->last_error = "You must pass an array to the update_array() function.";
return false;
}
$sql = "UPDATE $table SET";
foreach ($data as $key=>$value) {
$sql .= " $key=";
$col_type = $this->get_column_type($table, $key);
if (!$col_type) return false;
if (is_null($value)) {
$sql .= "NULL,";
}
elseif (substr_count(MYSQL_TYPES_NUMERIC, "$col_type ")) {
$sql .= "$value,";
}
elseif (substr_count(MYSQL_TYPES_DATE, "$col_type ")) {
$value = $this->sql_date_format($value, $col_type);
$sql .= "'$value',";
}
elseif (substr_count(MYSQL_TYPES_STRING, "$col_type ")) {
if ($this->auto_slashes) $value = addslashes($value);
$sql .= "'$value',";
}
}
$sql = rtrim($sql, ',');
if (!empty($condition)) $sql .= " WHERE $condition";
return $this->update_sql($sql);
}
function execute_file ($file) {
if (!file_exists($file)) {
$this->last_error = "The file $file does not exist.";
return false;
}
$str = file_get_contents($file);
if (!$str) {
$this->last_error = "Unable to read the contents of $file.";
return false;
}
$this->last_query = $str;
$sql = explode(';', $str);
foreach ($sql as $query) {
if (!empty($query)) {
$r = mysql_query($query);
if (!$r) {
$this->last_error = mysql_error();
return false;
}
}
}
return true;
}
function get_column_type($table, $column) {
$r = mysql_query("SELECT $column FROM $table");
if (!$r) {
$this->last_error = mysql_error();
return false;
}
$ret = mysql_field_type($r, 0);
if (!$ret) {
$this->last_error = "Unable to get column information on $table.$column.";
mysql_free_result($r);
return false;
}
mysql_free_result($r);
return $ret;
}
function sql_date_format($value) {
if (gettype($value) == 'string') $value = strtotime($value);
return date('Y-m-d H:i:s', $value);
}
function print_last_error($show_query=true) {
?>
<div style="border: 1px solid red; font-size: 9pt; font-family: monospace; color: red; padding: .5em; margin: 8px; background-color: #FFE2E2">
<span style="font-weight: bold">db.class.php Error:</span><br><?= $this->last_error ?>
</div>
<?
if ($show_query && (!empty($this->last_query))) {
$this->print_last_query();
}
}
public function get_link_id() {
return $this->link_id;
}
function print_last_query() {
?>
<div style="border: 1px solid blue; font-size: 9pt; font-family: monospace; color: blue; padding: .5em; margin: 8px; background-color: #E6E5FF">
<span style="font-weight: bold">Last SQL Query:</span><br><?= str_replace("\n", '<br>', $this->last_query) ?>
</div>
<?
}
function filter($data) {
$this->connect();
$data = trim(htmlentities(strip_tags($data)));
if (get_magic_quotes_gpc())
$data = stripslashes($data);
$data = mysql_real_escape_string($data);
return $data;
}
function page () {
ob_start();
session_start('WebATS');
include ("inc/htmltags.php");
include ("inc/class.content.php");
$Content = new Content();
$Content->ShowContent(PAGE);
echo '<title>'.$title.' - '.utf8_decode(PAGENAME).'</title>';
include ("inc/footer.php");
}
function account ($data) {
if($data == 'createaccount'){
define('_PAGETYPEREGISTER_','active');
} elseif ($data == 'login'){
define('_PAGETYPELOGIN_','active');
}elseif($data == ''){
define('_PAGETYPEREGISTER_','active');
}elseif($data == 'forgot_password'){
define('_PAGETYPEFORGOT_','active');
}elseif($data == 'active'){
define('_PAGETYPEACTIVE_','active');
}
}
}
?>obs: a linha 397 é a ultima linha, que contem ?>
ERRO PHP Parse error: syntax error
em PHP
Postado
tenho que baixar alguma coisa?? não entendi muito bem direito
por exemplo, substituir @mysql_connect($this->server,$this->user,$this->pw,$new_link) por mysqli_connect(string $host, string $username, string $passwd, string $dbname) ?